오늘은 Ducks Pattern 에 대해서 알아보겠습니다.
이것은 훅도 아니고 API도 아니고 꼭 지켜야 하는건 아니지만 개발하던 사람들이 이렇게 하면 편하더라 라고 하는 것입니다. 그럼 우리가 만듯것을 Ducks Pattern에 맞게 바꺼보겠습니다.
src/redux/modules폴더를 만들고 안에
add1,add2,reducer,user 4개의 파일을 옮겨주세요
add1.js
const ADD = "ADD";
const SUB = "SUB";
export function add(index) {
return {
type: ADD,
index,
};
}
export function subn(index) {
return {
type: SUB,
index,
};
}
export default function reducer(previousState = [], action) {
if (action.type === ADD) {
previousState.push(action.index);
return previousState;
}
if (action.type === SUB) {
return previousState.filter((p) => p !== action.index);
}
return previousState;
}
이렇게 수정
이게 무엇이냐 결과부터말하면 action.js 파일을 지우는 것입니다. 즉 add1과 관련된건 싹다 add1.js에 몰아 넣는 것입니다. 그래서 action에 있는 add1과 관련된것들을 가져왔고 함수들은 다른 곳에서 불러다가 쓸수있으니까 export를 유지하고 변수들은 내부에서 만 쓰니까 export를 다지워 주었습니다.
이제 add2 user 이런것들도 이런 규칙에 맞게 고치겠습니다.
add2.js
const ADD2 = "ADD2";
const SUB2 = "SUB2";
export function add2(index) {
return {
type: ADD2,
index,
};
}
export function subn2(index) {
return {
type: SUB2,
index,
};
}
export default function reducer(previousState = [], action) {
if (action.type === ADD2) {
previousState.push(action.index);
return previousState;
}
if (action.type === SUB2) {
return previousState.filter((p) => p !== action.index);
}
return previousState;
}
user.js
const DATA = "DATA";
export function indata(data) {
return {
type: DATA,
data,
};
}
export function adduser() {
return async (dispatch) => {
const res = await [
{ name: "mark1", id: 1 },
{ name: "mark2", id: 2 },
{ name: "mark3", id: 3 },
{ name: "mark4", id: 4 },
{ name: "mark5", id: 5 },
];
dispatch(indata(res));
console.log("adduser");
};
}
export default function User(state = { data: [] }, action) {
if (action.type === DATA) {
return { ...state, data: action.data };
}
return state;
}

이렇게 바꺼주고 마지막으로 여기서 import 해다가 쓰는 곳으로 가서 경로를 수정해주겠습니다.
component/add.js 가서
import { add } from "../redux/modules/add1";
이렇게 수정해주세요
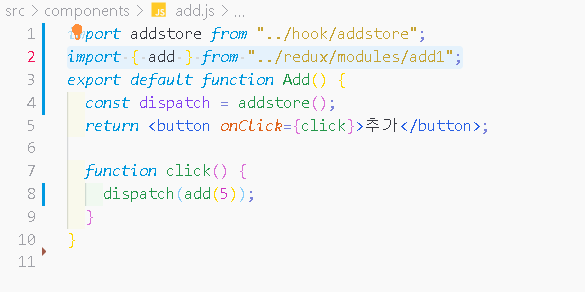
component/user.js가서
import { adduser } from "../redux/modules/user";
이렇게 수정 해주세요
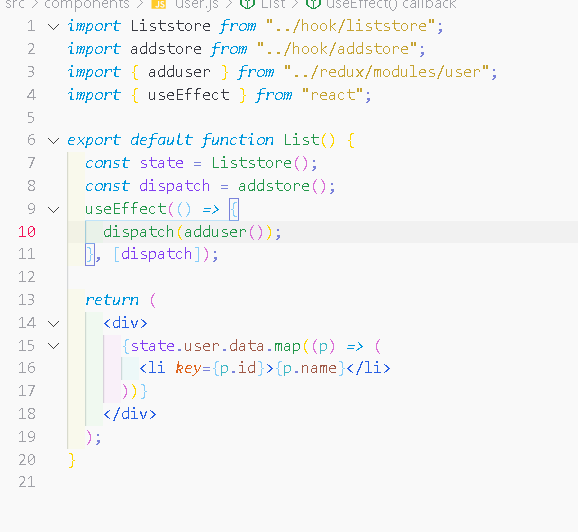
그러면 이제 제대로 동작되는지 확인해보겠습니다.
이렇게 제대로 동작 되는것을 보았습니다.
간단하게 오늘 배운것을 말하면 modules폴더만들고 그안에 관련된것들 모아서 파일로 만든다
정도로 생각할수 있습니다. ㅎㅎ
'React를 같이 배워보자' 카테고리의 다른 글
React23일차- 오늘은 쉬어가세요 ㅎㅎ (0) | 2022.02.12 |
---|---|
React22일차-promise and redux dev tools (0) | 2022.02.11 |
React21일차-middleware (0) | 2022.02.10 |
React21일차-Redux추가 (0) | 2022.02.10 |
React20일차-Redux를 react와 연결 (0) | 2022.02.09 |